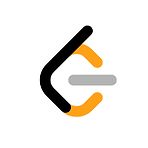
Roman numerals are represented by seven different symbols: I, V, X, L, C, D and M. Symbol Value I 1 V 5 X 10 L 50 C 100 D 500 M 1000 For example, two is written as II in Roman numeral, just two one's added together. Twelve is written as, XII, which is simply X + II. The number twenty seven is written as XXVII, which is XX + V + II. Roman numerals are usually written largest to smallest from left..
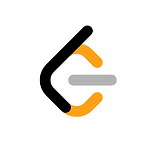
Determine whether an integer is a palindrome. An integer is a palindrome when it reads the same backward as forward. 정수가 회문인지 여부를 결정하십시오. 정수는 전방으로 동일한 것을 읽으면 회문입니다. /** * @param {number} x * @return {boolean} */ var isPalindrome = function(x) { return String(x) == String(x).split("").reverse().join(""); };
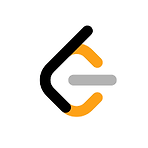
Given a 32-bit signed integer, reverse digits of an integer. - 주어진 32 비트 부호있는 정수, 정수의 역 자릿수. /** * @param {number} x * @return {number} */ var reverse = function(x) { var max = Math.pow(2, 31) -1, min = -1*(Math.pow(2, 31)); var r = 0, s = '', signed = 1; if(x >= max || x = max || r
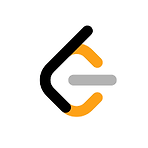
Given a string, find the length of the longest substring without repeating characters. 주어진 문자열에서 반복되는 문자없이 가장 긴 부분 문자열의 길이를 찾습니다. /** * @param {string} s * @return {number} */ var lengthOfLongestSubstring = function(s) { var r = '', t = '', st = 0; for(let i = 0 ; i < s.length ; i++){ let index = t.indexOf(s[i]); if(index != -1){ st = st + index + 1; } t = s.substring(st, i+1); if(r.length < t.l..
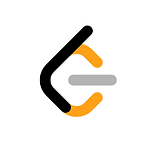
Given an array of integers, return indices of the two numbers such that they add up to a specific target. You may assume that each input would have exactly one solution, and you may not use the same element twice. 주어진 정수 배열을 이용하여 두 숫자의 인덱스를 반환하여 특정 대상에 합산합니다. 각 입력에는 정확히 하나의 솔루션이 있다고 가정 할 수 있으며 동일한 요소를 두 번 사용할 수 없습니다. /** * Definition for singly-linked list. * function ListNode(val) { * this.val ..
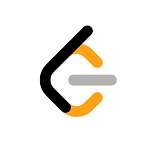
Given an array of integers, return indices of the two numbers such that they add up to a specific target. You may assume that each input would have exactly one solution, and you may not use the same element twice. 주어진 정수 배열을 이용하여 두 숫자의 인덱스를 반환하여 특정 대상에 합산합니다. 각 입력에는 정확히 하나의 솔루션이 있다고 가정 할 수 있으며 동일한 요소를 두 번 사용할 수 없습니다. /** * @param {number[]} nums * @param {number} target * @return {number[]} */ v..