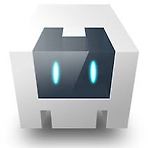
2달 간의 안드로이드 개발을 하고나서 느낀점을 기록 개발을 시작하게 된 계기 설연휴에 심심해서 한 번 해보고 싶어서 무엇을 만들지 부터 그리고 어떤 언어로 개발할지 가장 큰 고민 하이브리드 - 현재 내가 잘 할수 있는 언어로 한다(Javascript, Cordova), 필자는 포토샵을 조금 할줄암 사용자 반응성을 테스트 해보고 싶을 땐 가장 빨리 개발 할 수 있는 방향으로 설정 구글 API의 사용성 때문에 약간 도입하기 싫은 부분도 있었음 네이티브 - 조금 시간이 걸리더라도 배운다 ( Java, Kotlin ) 네이티브를 처음에 하기 싫었던 이유는 레이아웃 구성 때문(하지만 익히고 나니 별거없음) 자바는 조금이나마 다룰줄 알았음 리액트 네이티브는 처음부터 제외시켰다( 본인은 Vue를 사용하기 때문 ) i..
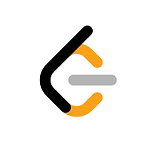
Given a sorted array and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order. You may assume no duplicates in the array. Example 1: Input: [1,3,5,6], 5 Output: 2 Example 2: Input: [1,3,5,6], 2 Output: 1 Example 3: Input: [1,3,5,6], 7 Output: 4 Example 4: Input: [1,3,5,6], 0 Output: 0 /** * @param {number[]} nums * @para..
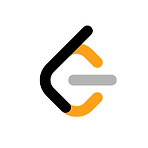
Write an algorithm to determine if a number is "happy". A happy number is a number defined by the following process: Starting with any positive integer, replace the number by the sum of the squares of its digits, and repeat the process until the number equals 1 (where it will stay), or it loops endlessly in a cycle which does not include 1. Those numbers for which this process ends in 1 are happ..
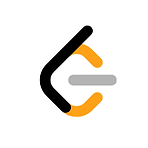
Given a sorted array nums, remove the duplicates in-place such that each element appear only once and return the new length. Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory. Example 1: Given nums = [1,1,2], Your function should return length = 2, with the first two elements of nums being 1 and 2 respectively. It doesn't..
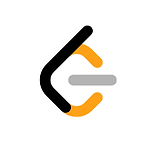
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists. 정렬 된 두 개의 링크 된 목록을 병합하고 새 목록으로 반환하십시오. 새 목록은 처음 두 목록의 노드를 서로 연결하여 만들어야합니다. /** * Definition for singly-linked list. * function ListNode(val) { * this.val = val; * this.next = null; * } */ /** * @param {ListNode} l1 * @param {ListNode} l2 * @return {ListNod..